手把手教您Android 自定义 ViewGroup
今天给大家带来一篇自定义ViewGroup的教程,说白了,就是教大家如何自定义ViewGroup,如果你对自定义ViewGroup还不是很了解,或者正想学习如何自定义,那么你可以好好看看这篇博客。
1、概述
在写代码之前,我必须得问几个问题:
1、ViewGroup的职责是啥?
ViewGroup相当于一个放置View的容器,并且我们在写布局xml的时候,会告诉容器(凡是以layout为开头的属性,都是为用于告诉容器的),我们的宽度(layout_width)、高度(layout_height)、对齐方式(layout_gravity)等;当然还有margin等;于是乎,ViewGroup的职能为:给childView计算出建议的宽和高和测量模式 ;决定childView的位置;为什么只是建议的宽和高,而不是直接确定呢,别忘了childView宽和高可以设置为wrap_content,这样只有childView才能计算出自己的宽和高。
2、View的职责是啥?
View的职责,根据测量模式和ViewGroup给出的建议的宽和高,计算出自己的宽和高;同时还有个更重要的职责是:在ViewGroup为其指定的区域内绘制自己的形态。
3、ViewGroup和LayoutParams之间的关系?
大家可以回忆一下,当在LinearLayout中写childView的时候,可以写layout_gravity,layout_weight属性;在RelativeLayout中的childView有layout_centerInParent属性,却没有layout_gravity,layout_weight,这是为什么呢?这是因为每个ViewGroup需要指定一个LayoutParams,用于确定支持childView支持哪些属性,比如LinearLayout指定LinearLayout.LayoutParams等。如果大家去看LinearLayout的源码,会发现其内部定义了LinearLayout.LayoutParams,在此类中,你可以发现weight和gravity的身影。
2、View的3种测量模式
上面提到了ViewGroup会为childView指定测量模式,下面简单介绍下三种测量模式:
EXACTLY:表示设置了精确的值,一般当childView设置其宽、高为精确值、match_parent时,ViewGroup会将其设置为EXACTLY;
AT_MOST:表示子布局被限制在一个*大值内,一般当childView设置其宽、高为wrap_content时,ViewGroup会将其设置为AT_MOST;
UNSPECIFIED:表示子布局想要多大就多大,一般出现在AadapterView的item的heightMode中、ScrollView的childView的heightMode中;此种模式比较少见。
注:上面的每一行都有一个一般,意思上述不是*对的,对于childView的mode的设置还会和ViewGroup的测量mode有一定的关系;当然了,这是*篇自定义ViewGroup,而且*大部分情况都是上面的规则,所以为了通俗易懂,暂不深入讨论其他内容。
3、从API角度进行浅析
上面叙述了ViewGroup和View的职责,下面从API角度进行浅析。
View的根据ViewGroup传人的测量值和模式,对自己宽高进行确定(onMeasure中完成),然后在onDraw中完成对自己的绘制。
ViewGroup需要给View传入view的测量值和模式(onMeasure中完成),而且对于此ViewGroup的父布局,自己也需要在onMeasure中完成对自己宽和高的确定。此外,需要在onLayout中完成对其childView的位置的指定。
4、完整的例子
需求:我们定义一个ViewGroup,内部可以传入0到4个childView,分别依次显示在左上角,右上角,左下角,右下角。
1、决定该ViewGroup的LayoutParams
对于我们这个例子,我们只需要ViewGroup能够支持margin即可,那么我们直接使用系统的MarginLayoutParams
-
-
public ViewGroup.LayoutParams generateLayoutParams(AttributeSet attrs)
-
-
return new MarginLayoutParams(getContext(), attrs);
-
重写父类的该方法,返回MarginLayoutParams的实例,这样就为我们的ViewGroup指定了其LayoutParams为MarginLayoutParams。
2、onMeasure
在onMeasure中计算childView的测量值以及模式,以及设置自己的宽和高:
-
-
-
-
-
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec)
-
-
-
-
-
int widthMode = MeasureSpec.getMode(widthMeasureSpec);
-
int heightMode = MeasureSpec.getMode(heightMeasureSpec);
-
int sizeWidth = MeasureSpec.getSize(widthMeasureSpec);
-
int sizeHeight = MeasureSpec.getSize(heightMeasureSpec);
-
-
-
-
measureChildren(widthMeasureSpec, heightMeasureSpec);
-
-
-
-
-
-
-
int cCount = getChildCount();
-
-
-
-
MarginLayoutParams cParams = null;
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
for (int i = 0; i < cCount; i++)
-
-
View childView = getChildAt(i);
-
cWidth = childView.getMeasuredWidth();
-
cHeight = childView.getMeasuredHeight();
-
cParams = (MarginLayoutParams) childView.getLayoutParams();
-
-
-
-
-
tWidth += cWidth + cParams.leftMargin + cParams.rightMargin;
-
-
-
-
-
bWidth += cWidth + cParams.leftMargin + cParams.rightMargin;
-
-
-
-
-
lHeight += cHeight + cParams.topMargin + cParams.bottomMargin;
-
-
-
-
-
rHeight += cHeight + cParams.topMargin + cParams.bottomMargin;
-
-
-
-
-
width = Math.max(tWidth, bWidth);
-
height = Math.max(lHeight, rHeight);
-
-
-
-
-
-
setMeasuredDimension((widthMode == MeasureSpec.EXACTLY) ? sizeWidth
-
: width, (heightMode == MeasureSpec.EXACTLY) ? sizeHeight
-
-
10-14行,获取该ViewGroup父容器为其设置的计算模式和尺寸,大多情况下,只要不是wrap_content,父容器都能正确的计算其尺寸。所以我们自己需要计算如果设置为wrap_content时的宽和高,如何计算呢?那就是通过其childView的宽和高来进行计算。
17行,通过ViewGroup的measureChildren方法为其所有的孩子设置宽和高,此行执行完成后,childView的宽和高都已经正确的计算过了
43-71行,根据childView的宽和高,以及margin,计算ViewGroup在wrap_content时的宽和高。
80-82行,如果宽高属性值为wrap_content,则设置为43-71行中计算的值,否则为其父容器传入的宽和高。
3、onLayout对其所有childView进行定位(设置childView的绘制区域)
-
-
-
protected void onLayout(boolean changed, int l, int t, int r, int b)
-
-
int cCount = getChildCount();
-
-
-
MarginLayoutParams cParams = null;
-
-
-
-
for (int i = 0; i < cCount; i++)
-
-
View childView = getChildAt(i);
-
cWidth = childView.getMeasuredWidth();
-
cHeight = childView.getMeasuredHeight();
-
cParams = (MarginLayoutParams) childView.getLayoutParams();
-
-
int cl = 0, ct = 0, cr = 0, cb = 0;
-
-
-
-
-
-
-
-
-
cl = getWidth() – cWidth – cParams.leftMargin
-
-
-
-
-
-
-
ct = getHeight() – cHeight – cParams.bottomMargin;
-
-
-
cl = getWidth() – cWidth – cParams.leftMargin
-
-
ct = getHeight() – cHeight – cParams.bottomMargin;
-
-
-
-
-
-
childView.layout(cl, ct, cr, cb);
-
-
-
代码比较容易懂:遍历所有的childView,根据childView的宽和高以及margin,然后分别将0,1,2,3位置的childView依次设置到左上、右上、左下、右下的位置。
如果是*个View(index=0) :则childView.layout(cl, ct, cr, cb); cl为childView的leftMargin , ct 为topMargin , cr 为cl+ cWidth , cb为 ct + cHeight
如果是第二个View(index=1) :则childView.layout(cl, ct, cr, cb);
cl为getWidth() – cWidth – cParams.leftMargin- cParams.rightMargin;
ct 为topMargin , cr 为cl+ cWidth , cb为 ct + cHeight
剩下两个类似~
这样就完成了,我们的ViewGroup代码的编写,下面我们进行测试,分别设置宽高为固定值,wrap_content,match_parent
5、测试结果
布局1:
-
<com.example.zhy_custom_viewgroup.CustomImgContainer xmlns:android=“http://schemas.android.com/apk/res/android”
-
xmlns:tools=“http://schemas.android.com/tools”
-
android:layout_width=“200dp”
-
android:layout_height=“200dp”
-
android:background=“#AA333333” >
-
-
-
android:layout_width=“50dp”
-
android:layout_height=“50dp”
-
android:background=“#FF4444”
-
-
-
android:textColor=“#FFFFFF”
-
-
android:textStyle=“bold” />
-
-
-
android:layout_width=“50dp”
-
android:layout_height=“50dp”
-
android:background=“#00ff00”
-
-
-
android:textColor=“#FFFFFF”
-
-
android:textStyle=“bold” />
-
-
-
android:layout_width=“50dp”
-
android:layout_height=“50dp”
-
android:background=“#ff0000”
-
-
-
android:textColor=“#FFFFFF”
-
-
android:textStyle=“bold” />
-
-
-
android:layout_width=“50dp”
-
android:layout_height=“50dp”
-
android:background=“#0000ff”
-
-
-
android:textColor=“#FFFFFF”
-
-
android:textStyle=“bold” />
-
-
</com.example.zhy_custom_viewgroup.CustomImgContainer>
ViewGroup宽和高设置为固定值
效果图:
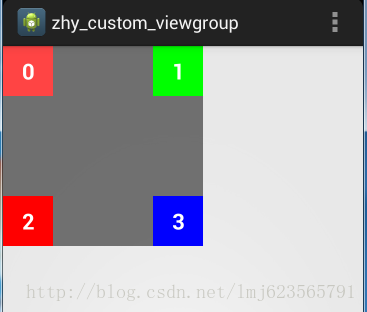
布局2:
-
<com.example.zhy_custom_viewgroup.CustomImgContainer xmlns:android=“http://schemas.android.com/apk/res/android”
-
xmlns:tools=“http://schemas.android.com/tools”
-
android:layout_width=“wrap_content”
-
android:layout_height=“wrap_content”
-
android:background=“#AA333333” >
-
-
-
android:layout_width=“150dp”
-
android:layout_height=“150dp”
-
android:background=“#E5ED05”
-
-
-
android:textColor=“#FFFFFF”
-
-
android:textStyle=“bold” />
-
-
-
android:layout_width=“50dp”
-
android:layout_height=“50dp”
-
android:background=“#00ff00”
-
-
-
android:textColor=“#FFFFFF”
-
-
android:textStyle=“bold” />
-
-
-
android:layout_width=“50dp”
-
android:layout_height=“50dp”
-
android:background=“#ff0000”
-
-
-
android:textColor=“#FFFFFF”
-
-
android:textStyle=“bold” />
-
-
-
android:layout_width=“50dp”
-
android:layout_height=“50dp”
-
android:background=“#0000ff”
-
-
-
android:textColor=“#FFFFFF”
-
-
android:textStyle=“bold” />
-
-
</com.example.zhy_custom_viewgroup.CustomImgContainer>
ViewGroup的宽和高设置为wrap_content
效果图:
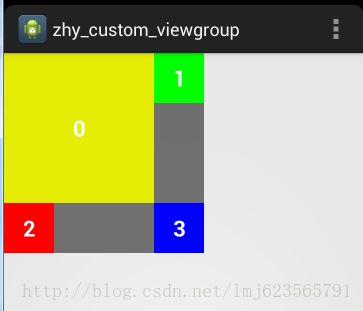
布局3:
-
<com.example.zhy_custom_viewgroup.CustomImgContainer xmlns:android=“http://schemas.android.com/apk/res/android”
-
xmlns:tools=“http://schemas.android.com/tools”
-
android:layout_width=“match_parent”
-
android:layout_height=“match_parent”
-
android:background=“#AA333333” >
-
-
-
android:layout_width=“150dp”
-
android:layout_height=“150dp”
-
android:background=“#E5ED05”
-
-
-
android:textColor=“#FFFFFF”
-
-
android:textStyle=“bold” />
-
-
-
android:layout_width=“50dp”
-
android:layout_height=“50dp”
-
android:background=“#00ff00”
-
-
-
android:textColor=“#FFFFFF”
-
-
android:textStyle=“bold” />
-
-
-
android:layout_width=“50dp”
-
android:layout_height=“50dp”
-
android:background=“#ff0000”
-
-
-
android:textColor=“#FFFFFF”
-
-
android:textStyle=“bold” />
-
-
-
android:layout_width=“150dp”
-
android:layout_height=“150dp”
-
android:background=“#0000ff”
-
-
-
android:textColor=“#FFFFFF”
-
-
android:textStyle=“bold” />
-
-
</com.example.zhy_custom_viewgroup.CustomImgContainer>
ViewGroup的宽和高设置为match_parent
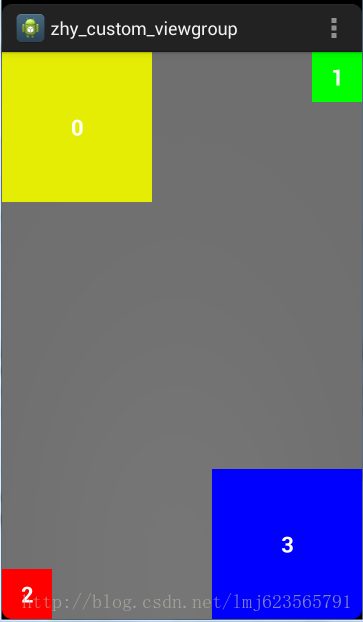
可以看到无论ViewGroup的宽和高的值如何定义,我们的需求都实现了预期的效果~~
好了,通过这篇教程,希望大家已经能够初步掌握自定义ViewGroup的步骤,大家可以尝试自定义ViewGroup去实现LinearLayout的效果~~